Given an array, the task is to insert an element at a specific position in an array in JavaScript.
Given Array arr = [ 10, 20, 30, 40, 50 ]
Element = 25, Position = 2
Updated Array arr = [ 10, 20, 25, 30, 40, 50 ]
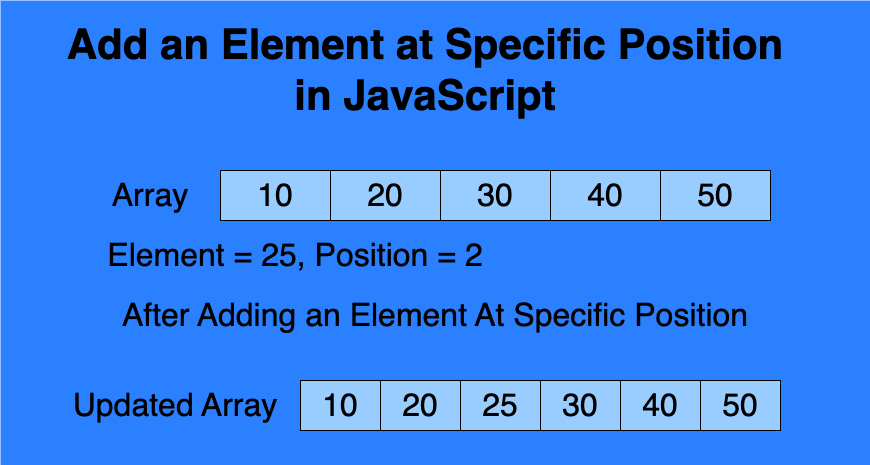
There are multiple approaches to add an element at a specific position in JavaScript.
1. Using splice() Method
The splice()
method is used to insert an element at a specific position in an array. This method allows you to add, remove, or replace elements.
array.splice(start, deleteCount, item1, item2, ..., itemN);
Example
let arr = [ 10, 20, 30, 40, 50 ];
arr.splice(2, 0, 25);
console.log(arr); // Output: [ 10, 20, 25, 30, 40, 50 ]
Features
- Time Complexity: O(n) (due to array shifting).
- Modifies Original Array: Yes.
2. Using Spread Operator (…)
The spread operator works with splice() method to create a new array by spreading elements before and after the insertion point, while adding the new element at the desired position.
let newArray = [...array.slice(0, position), element, ...array.slice(position)];
Example
let arr = [ 10, 20, 30, 40, 50 ];
let updatedArr = [...arr.slice(0, 2), 25, ...arr.slice(2)];
console.log(updatedArr); // Output: [ 10, 20, 25, 30, 40, 50 ]
Features
- Time Complexity: O(n) (due to array slicing and spreading).
- Modifies Original Array: No.
3. Using concat() Method
The concat()
method creates a new array by merging multiple arrays or elements. This method can be used to to insert elements at a specific position by concatenating slices of the original array.
let newArray = [].concat(array.slice(0, position), element, array.slice(position));
Example
let arr = [ 10, 20, 30, 40, 50 ];
let updatedArr = [].concat(arr.slice(0, 2), 25, arr.slice(2));
console.log(updatedArr); // Output: [ 10, 20, 25, 30, 40, 50 ]
Features
- Time Complexity: O(n) (due to slicing and concatenation).
- Modifies Original Array: No.
4. Using Array Indexing and Manual Shifting
We can manually shift elements starting from the target position to make room for the new element.
Example
let arr = [ 10, 20, 30, 40, 50 ];
let position = 2;
let newElement = 25;
for (let i = arr.length; i > position; i--) {
arr[i] = arr[i - 1];
}
arr[position] = newElement;
console.log(arr); // Output: [ 10, 20, 25, 30, 40, 50 ]
Features
- Time Complexity: O(n) (due to element shifting).
- Modifies Original Array: Yes.