Given an Array, the task is to add an element to the end of an array in JavaScript.
Input Array arr = [ 10, 20, 30, 40, 50 ]
Item = 60
Updated Array: [ 10, 20, 30, 40, 50, 60 ]
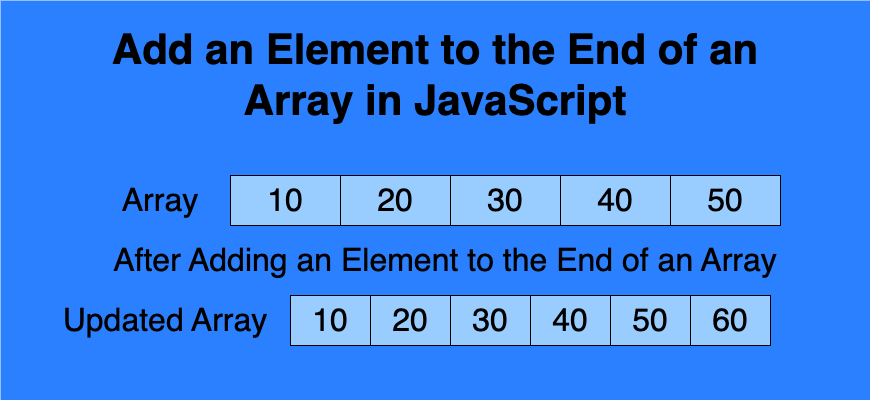
There are multiple ways to add an element to the end of an array in JavaScript. These are –
1. Using push() Method
The push()
method is a very basic method to add an element to the end of an array. This method directly modifies the original array and appends one or more elements.
array.push(element1, element2, ..., elementN);
Example
let arr = [ 10, 20, 30, 40, 50 ]
arr.push(60);
console.log(arr); // Output: [ 10, 20, 30, 40, 50, 60 ]
Features
- Time Complexity: O(1) (constant time).
- Modifies Original Array: Yes.
2. Using Spread Operator (…)
The spread operator can also be used to add elements to the end of an array. It creates a new array by spreading the elements of the original array and appending the new element.
let newArray = [...array, element];
Example
let arr = [ 10, 20, 30, 40, 50 ]
let updatedArr = [...arr, 60];
console.log(updatedArr); // Output: [ 10, 20, 30, 40, 50, 60 ]
Features
- Time Complexity: O(n) (linear time, as it creates a new array).
- Modifies Original Array: No.
3. Using concat() Method
The concat()
method creates a new array by merging the original array with one or more additional values.
let newArray = array.concat(element1, element2, ..., elementN);
Example
let arr = [ 10, 20, 30, 40, 50 ]
let updatedArr = arr.concat(60);
console.log(updatedArr); // Output: [ 10, 20, 30, 40, 50, 60 ]
Features
- Time Complexity: O(n).
- Modifies Original Array: No.
4. Using Array Indexing
You can directly assign a value to the index equal to the current length of the array.
array[array.length] = element;
Example
let arr = [ 10, 20, 30, 40, 50 ]
arr[arr.length] = 60;
console.log(arr); // Output: [ 10, 20, 30, 40, 50, 60 ]
Features
- Time Complexity: O(1).
- Modifies Original Array: Yes.
5. Using splice() Method
The splice()
method is used to add, remove, or replace elements in an array. This method append an element, specify the insertion point as the array’s current length.
array.splice(start, deleteCount, item1, item2, ..., itemN);
Example
let arr = [ 10, 20, 30, 40, 50 ]
arr.splice(arr.length, 0, 60);
console.log(arr); // Output: [ 10, 20, 30, 40, 50, 60 ]
Features
- Time Complexity: O(n) (due to potential array shifting).
- Modifies Original Array: Yes.
6. Handling Edge Cases
Adding Multiple Elements
Some methods like push()
and concat()
can also be used to add multiple elements at once.
Example
let arr = [ 10, 20, 30, 40, 50 ]
arr.push(60, 70, 80);
console.log(arr);
/* Output: [
10, 20, 30, 40,
50, 60, 70, 80
] */
Adding Elements to an Empty Array
All methods work the same way with empty arrays.
Example
let arr = [];
arr.push(10, 20, 30, 40);
console.log(arr); // Output: [ 10, 20, 30, 40 ]
Adding Non-Primitive Elements
Arrays, objects, and functions can also be appended using above approaches.
Example
let arr = [10, 20];
arr.push([30, 40]);
console.log(arr); // Output: [ 10, 20, [ 30, 40 ] ]