HTML is the basic building block of any website. If you want to start your career in web development then it is mandatory to learn HTML for basic understanding. In this article, we will create first HTML project to create a simple HTML page.
A simple HTML page contains some basic HTML elements like <html>, <head>, <body>, … etc. CSS is also required to style the webpage.
Basic Structure of HTML Document
<!DOCTYPE html> <html lang="en"> <head> <title>Basic HTML Page</title> </head> <body> <h1>Heading of Web Page</h1> <p>Paragraph Content...</p> </body> </html>
The <head> tag contains the meta information and page title. The contents under <body> tag is visible to the browser window.
Design a Simple HTML Page
To design the simple HTML page, we will add some heading, paragraph, and image to the web page.
<h1>Simple HTML Page</h1> <p> It is the first project of HTML. This page contains heading, paragraph, and image. </p> <img src="logo.png" alt="Logo Image">
After adding the HTML code, we need to add some CSS styles to make the webpage better.
/* Setting the padding and margin of webpage to 0 */ * { padding: 0; margin: 0; box-sizing: border-box; } /* Setting the font style, background color and text alignment */ body { font-family: 'Times New Roman', Times, serif; background-color: aqua; text-align: center; } /* Setting the image size */ img { width: 300px; height: 200px; margin-top: 50px; }
After combining the HTML, and CSS code, we will get the complete code of simple HTML page.
<!DOCTYPE html> <html lang="en"> <head> <title>Design a Simple HTML Page</title> <style> * { padding: 0; margin: 0; box-sizing: border-box; } body { font-family: 'Times New Roman', Times, serif; background-color: aqua; text-align: center; } img { width: 300px; height: 200px; margin-top: 50px; } </style> </head> <body> <h1>Simple HTML Page</h1> <p> It is the first project of HTML. This page contains heading, paragraph, and image. </p> <img src="logo.png" alt="Logo image"> </body> </html>
Output
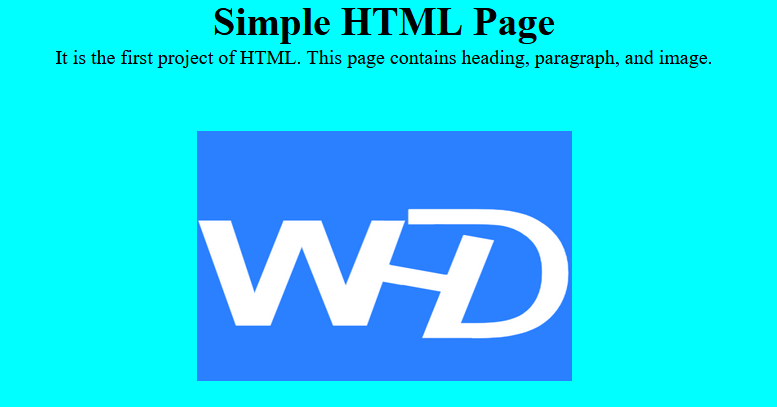
You can practice and create a basic HTML page with different HTML elements and CSS styles.