A two-dimensional array is an array which contain another arrays as an array elements. The 2D array commonly used to represent grids, matrices, or tabular data.
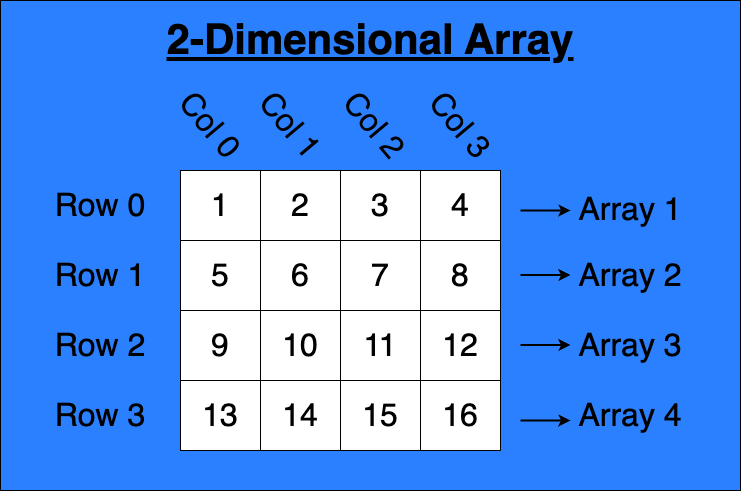
What is a Two-Dimensional Array?
A two-dimensional array is an array where each element is an array itself. It represents array data in tabular format like rows and columns.
let twoDArray = [
[1, 2, 3], // Row 1
[4, 5, 6], // Row 2
[7, 8, 9] // Row 3
];
- The main array contains three arrays (rows).
- Each sub-array contains three elements (columns).
Create a Two-Dimensional Array
It is a basic method to create and initialise an array. First, we declare an array, and initialise the array using array elements. Two-Dimensional array contains array items in form of array of arrays.
let twoDArr = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; console.log(twoDArr); /* Output: [ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ] */
Create a Two-Dimensional Array Dynamically
The dynamic method to create a two-dimensional array is useful while the size of array is not given.
1. Using for Loops
You can use nested loops to create and initialize a two-dimensional array.
Example: Creating a 2D Array with some values
let rows = 3; let cols = 4; // Declare an empty array let twoDArr = []; for (let i = 0; i < rows; i++) { twoDArr[i] = []; for (let j = 0; j < cols; j++) { twoDArr[i][j] = i+j; // Initialize values } } console.log(twoDArr); /* Output: [ [ 0, 1, 2, 3 ], [ 1, 2, 3, 4 ], [ 2, 3, 4, 5 ] ] */
2. Using Array.from() Method
The Array.from()
method creates an array and apply a mapping function to initialize the array.
Example: Empty 2D Array
let rows = 3; let cols = 4; let twoDArr = Array.from({ length: rows }, () => Array.from({ length: cols }, () => 0)); console.log(twoDArr); /* Output: [ [ 0, 0, 0, 0 ], [ 0, 0, 0, 0 ], [ 0, 0, 0, 0 ] ] */
Example: Creating a 2D array with Predefined Values
let rows = 3; let cols = 4; let twoDArr = Array.from({ length: rows }, (_, i) => Array.from({ length: cols }, (_, j) => i + j) ); console.log(twoDArr); /* Output: [ [ 0, 1, 2, 3 ], [ 1, 2, 3, 4 ], [ 2, 3, 4, 5 ] ] */
3. Using fill() Method
The fill()
method can also be used to populate an array with identical values. For two-dimensional arrays, you need nested calls.
let rows = 3; let cols = 4; let twoDArr = new Array(rows).fill() .map(() => new Array(cols).fill(0)); console.log(twoDArr); /* Output: [ [ 0, 0, 0, 0 ], [ 0, 0, 0, 0 ], [ 0, 0, 0, 0 ] ] */
How It Works?
new Array(rows).fill()
creates an array of the desired number of rows.map()
initializes each row with an array of zeros.
4. Using Array Destructuring
The array destructuring initializes a 2D array dynamically.
let rows = 3; let cols = 4; let twoDArr = [...Array(rows)].map(() => Array(cols).fill(0)); console.log(twoDArr); /* Output: [ [ 0, 0, 0, 0 ], [ 0, 0, 0, 0 ], [ 0, 0, 0, 0 ] ] */
Sparse Two-Dimensional Arrays
A sparse array is a two-dimensional array where only specific elements are initialized, leaving other elements undefined.
let sparseArray = []; sparseArray[0] = []; sparseArray[0][1] = 42; console.log(sparseArray); // Output: [ [ <1 empty item>, 42 ] ]