JavaScript 2D Array (Two-Dimensional Array in JavaScript) is a data structure that is used to store elements in a grid-like fashion using rows and columns. It can be visualized as an array of arrays, where each inner array represents a row. 2D arrays are useful in scenarios like representing matrices, tables, or grids in a game.
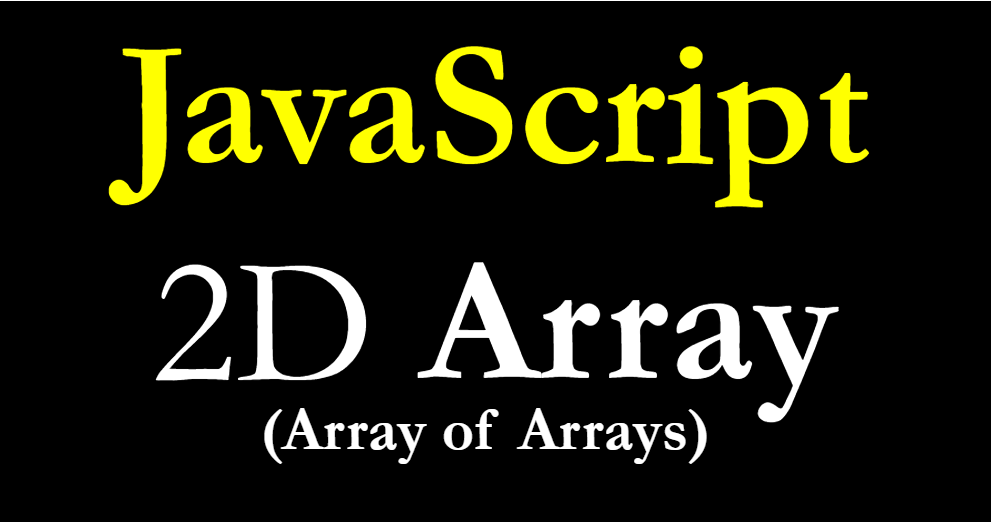
Creating a 2D Array in JavaScript
There are multiple ways to create a 2D array in JavaScript. Here are two common approaches:
1. Manually Initializing a 2D Array
Creating a 3×3 matrix where each row is represented by an inner array.
const mat = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; console.log(mat);
Output
[ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ]
2. Using Nested Loops to Populate a 2D Array
Dynamically create a 2D array using nested loops and populate it with sequential numbers.
const rows = 3; const cols = 3; const mat = []; for (let i = 0; i < rows; i++) { const row = []; for (let j = 0; j < cols; j++) { // Populate with sequential numbers row.push(i * cols + j + 1); } mat.push(row); } console.log(mat);
Output
[ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ]
Accessing Elements in a 2D Array
To access elements in a 2D array, you can use the row and column indices. JavaScript uses 0-based indexing, meaning the first row and column start at index 0.
const mat = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; console.log(mat[0][0]); console.log(mat[1][2]); console.log(mat[2][1]);
Output
1
6
8
Modifying Elements in a 2D Array
You can modify elements in a 2D array by assigning new values using their row and column indices.
const mat = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; // Change the second element of the first row mat[0][1] = 20; console.log(mat); // Change the last element of the last row mat[2][2] = 99; console.log(mat);
Output
[ [ 1, 20, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ]
[ [ 1, 20, 3 ], [ 4, 5, 6 ], [ 7, 8, 99 ] ]
Iterating Over a 2D Array
To process all elements in a 2D array, you can use nested loops.
const mat = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; for (let i = 0; i < mat.length; i++) { for (let j = 0; j < mat[i].length; j++) { console.log(`Element at (${i}, ${j}): ${mat[i][j]}`); } }
Output
Element at (0, 0): 1
Element at (0, 1): 2
Element at (0, 2): 3
Element at (1, 0): 4
Element at (1, 1): 5
Element at (1, 2): 6
Element at (2, 0): 7
Element at (2, 1): 8
Element at (2, 2): 9
Common Use Cases for 2D Arrays
1. Representing a Matrix
2D arrays are ideal for representing mathematical matrices. For example:
const matA = [ [1, 2], [3, 4] ]; const matB = [ [5, 6], [7, 8] ]; // Adding matrices const result = []; for (let i = 0; i < matA.length; i++) { const row = []; for (let j = 0; j < matA[i].length; j++) { row.push(matA[i][j] + matB[i][j]); } result.push(row); } console.log(result);
Output
[ [ 6, 8 ], [ 10, 12 ] ]
2. Game Boards
A 2D array can represent a game board, such as a chessboard or tic-tac-toe grid.
const ticTacToe = [ ['X', 'O', 'X'], ['O', 'X', 'O'], ['X', 'O', ''] ]; console.log(ticTacToe);
Output
[ [ 'X', 'O', 'X' ], [ 'O', 'X', 'O' ], [ 'X', 'O', '' ] ]
3. Tables or Grids
2D arrays can store data from a table or grid, such as a spreadsheet.
const table = [ ['Name', 'Age', 'City'], ['Alice', 25, 'New York'], ['Bob', 30, 'San Francisco'], ['Charlie', 35, 'Los Angeles'] ]; console.log(table);
Output
[
[ 'Name', 'Age', 'City' ],
[ 'Alice', 25, 'New York' ],
[ 'Bob', 30, 'San Francisco' ],
[ 'Charlie', 35, 'Los Angeles' ]
]
Converting Between 1D and 2D Arrays
Flattening a 2D Array to 1D Array
You can convert a 2D array into a 1D array using methods like Array.prototype.flat()
.
const mat = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; const flatArray = mat.flat(); console.log(flatArray);
Output
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Creating a 2D Array from a 1D Array
You can convert a 1D array into a 2D array by grouping elements.
const flatArray = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const cols = 3; const mat = []; for (let i = 0; i < flatArray.length; i += cols) { mat.push(flatArray.slice(i, i + cols)); } console.log(mat);
Output
[ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ]