Given an array with elements, the task is to remove the last element of an array in JavaScript.
Input: arr = [ 10, 20, 30, 40, 50 ]
After removing the last element
Output: Updated Array: [ 10, 20, 30, 40 ]
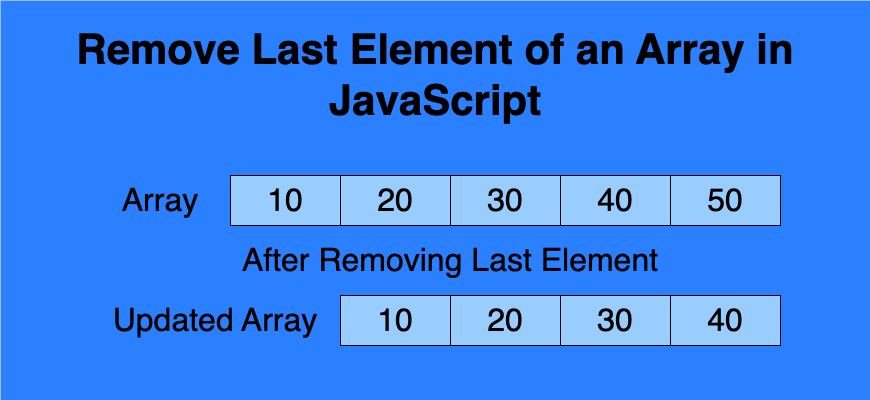
1. Using pop() Method
The pop()
method is a basic and most used approach to remove the last element from an array. This method modifies the original array and returns the removed element.
array.pop();
Example
let arr = [ 10, 20, 30, 40, 50 ];
let removedElement = arr.pop();
console.log(arr); // Output: [ 10, 20, 30, 40 ]
console.log(removedElement); // Output: 50
Features
- Time Complexity: O(1).
- Modifies Original Array: Yes.
- Returns: The removed element.
2. Using slice() Method
The slice()
method creates a new array excluding the last element by slicing from the beginning to the second-to-last index. It doesn’t modify the original array.
let newArray = array.slice(0, -1);
Example
let arr = [ 10, 20, 30, 40, 50 ];
let newArr = arr.slice(0, -1);
console.log(newArr); // Output: [ 10, 20, 30, 40 ]
Features
- Time Complexity: O(n) (depends on the array size).
- Modifies Original Array: No.
- Returns: A new array with the last element removed.
3. Using splice() Method
The splice()
method removes one or more elements from an array at a specified position. To remove the last element, set the starting index to array.length - 1
and the delete count to 1
.
array.splice(start, deleteCount);
Example
let arr = [ 10, 20, 30, 40, 50 ];
let removedElement = arr.splice(arr.length - 1, 1);
console.log(arr); // [ 10, 20, 30, 40 ]
console.log(removedElement); // Output: [ 50 ]
Features
- Time Complexity: O(1) for removing the last element.
- Modifies Original Array: Yes.
- Returns: An array containing the removed element(s).
4. Using length Property
The length
property can also be used to remove the last element of an array. This method directly modifies the original array.
array.length = array.length - 1;
Example
let arr = [ 10, 20, 30, 40, 50 ];
arr.length = arr.length - 1;
console.log(fruits); // Output: [ 10, 20, 30, 40 ]
Features
- Time Complexity: O(1).
- Modifies Original Array: Yes.
- Returns: Nothing.
5. Using filter() Method
The filter()
method creates a new array containing all elements except the last one by filtering out the element at the last index.
let newArray = array.filter((_, index) => index < array.length - 1);
Example
let arr = [ 10, 20, 30, 40, 50 ];
let newArr = arr.filter((_, index) => index < arr.length - 1);
console.log(arr); // Output: [ 10, 20, 30, 40, 50 ]
console.log(newArr); // Output: [ 10, 20, 30, 40 ]
Features
- Time Complexity: O(n).
- Modifies Original Array: No.
- Returns: A new array with the last element removed.
Edge Cases
1. Removing the Last Element from an Empty Array
Using pop()
method on an empty array returns undefined
and does nothing to the array.
let arr = [];
let removedElement = arr.pop();
console.log(arr); // Output: []
console.log(removedElement); // Output: undefined
2. Removing the Last Element of a Single-Element Array
All methods work seamlessly:
let arr = [ 10 ];
arr.pop();
console.log(arr); // Output: []